ReactNativeとは
- Viewをコンポーネント単位で表示するためのライブラリ
- Javascriptで書いて、ネイティブのViewでレンダリングされるため高速
実現したいこと
- Appleの審査を待たずにアプリをバージョンアップさせたい
- 一定のパフォーマンスは保ちたい
- アプリ全体ではなく、一部分の置き換え
前提
- 既存システムをReactNativeで置き換える
- ほとんどネイティブの機能を使っていない
検討
- 実はwebviewでもよいかも、パフォーマンスの比較もしたい
導入
- http://www.reactnative.com/
- http://facebook.github.io/react-native/docs/getting-started.html#content
brew install node brew install watchman brew install flow
npm install -g react-native-cli
サンプルプロジェクトの作成
react-native init AwesomeProject
AwesomeProjectディレクトリがつくられる
- AwesomeProject.xcodeproj
- iOS
- node_modules
- package.json起動
Xcodeを立ち上げて、⌘+Rでいつも通り起動サンプルプロジェクトでは、ビルド時にnodeを起動している
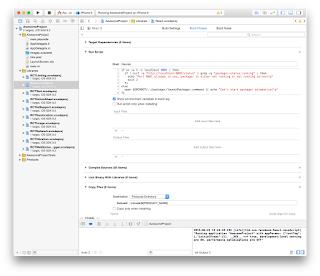
レンダリングするjsを指定
レンダリングするjsはアプリにインストールしたファイルからも、webからも取得することが可能// webから取得する場合
jsCodeLocation = [NSURL URLWithString:@"http://localhost:8081/index.ios.bundle"];
// アプリ内のファイルを使う場合
jsCodeLocation = [[NSBundle mainBundle] URLForResource:@"main" withExtension:@"jsbundle"];
// 描画させる
RCTRootView *rootView = [[RCTRootView alloc] initWithBundleURL:jsCodeLocation
moduleName:@"AwesomeProject"
launchOptions:launchOptions];
アプリ内にファイルを置く場合は以下のコマンドで取得$ curl 'http://localhost:8081/index.ios.bundle?dev=false&minify=true' -o iOS/main.jsbundle
Cmd + Rで再読み込みできない場合
Simulator の Hardware > keyboard の設定を確認既存プロジェクトへの導入
CocoaPods
pod 'React'
pod 'React/RCTText'
Bridge-Header.hの追加#import <RCTRootView.h>
iOS App
ViewControllerのviewなどにコードから追加する@IBOutlet weak var wrapView: UIView!
var rootView:RCTRootView? = nil
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
var jsCodeLocation = NSURL(string:"http://localhost:8081/index.ios.bundle")
rootView = RCTRootView(bundleURL: jsCodeLocation, moduleName: "SimpleApp", launchOptions: nil)
rootView!.frame = wrapView.bounds
wrapView.addSubview(rootView!)
}
React Native App作成
ReactComponentディレクトリを作り中身は index.ios.js を置く'use strict';
var React = require('react-native');
var {
Text,
View
} = React;
var styles = React.StyleSheet.create({
container: {
flex: 1,
backgroundColor: 'red'
}
});
class SimpleApp extends React.Component {
render() {
return (
<View style={styles.container}>
<Text>This is a simple application.</Text>
</View>
)
}
}
React.AppRegistry.registerComponent('SimpleApp', () => SimpleApp);
開発サーバの起動
(JS_DIR=`pwd`/ReactComponent; cd Pods/React; npm run start -- --root $JS_DIR)
デバッグ
RCTWebSocketDebuggerを追加すると⌘Rで更新ができるpod 'React/RCTWebSocketDebugger
⌘+Ctl+Zでデバッグメニューを表示させるには、シェイクジェスチャーのdelegateを呼ぶ必要があるoverride func motionEnded(motion: UIEventSubtype, withEvent event: UIEvent) {
rootView?.motionEnded(motion, withEvent: event)
}
0 件のコメント:
コメントを投稿